Como implementar a mascara do CEP ?
<form noValidate autoComplete='off' onSubmit={handleSubmit(onSubmit)}>
<FormControl fullWidth sx={{ mb: 4 }}>
<Controller
name='cep'
control={control}
rules={{ required: true }}
render={({ field: { value, onChange, onBlur } }) => (
<TextField
required
label='CEP'
value={value}
onBlur={onBlur}
onChange={onChange}
error={Boolean(errors.cep)}
inputProps={{ maxLength: 9 }}
/>
)}
/>
{errors.cep && <FormHelperText sx={{ color: 'error.main' }}>{errors.cep.message}</FormHelperText>}
</FormControl>
</form>
export const mascaraCep = (value: string) => {
if (!value) {
return ''
}
return value
.replace(/\D/g, '')
.replace(/^(\d{5})(\d{3})+?$/, '$1-$2')
.replace(/(-\d{3})(\d+?)/, '$1')
}
import React from "react";
import InputMask from "react-input-mask";
// import InputMask from "react-text-mask";
import { useForm, Controller } from "react-hook-form";
export default function App() {
const [user, setUser] = React.useState({});
const { register, handleSubmit, control } = useForm();
function onSubmit(data) {
console.log(data);
}
return (
<div className="App">
<form onSubmit={handleSubmit(onSubmit)}>
<input
type="text"
name="name"
placeholder="User full name"
ref={register}
defaultValue={user.name}
/>
<input
type="email"
name="email"
placeholder="User email"
ref={register}
defaultValue={user.email}
/>
<Controller
as={InputMask}
control={control}
mask="99999-999"
name="cep"
defaultValue={user.cep}
/>
<button type="submit">SEND</button>
</form>
</div>
);
}
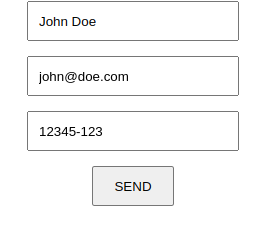
1 curtida
Este erro:
Type '{ as: typeof ReactInputMask; name: "cep"; mask: string; control: Control<{ cep: string; logradouro: string; numero: number; complemento: string; estado: string; bairro: string; cidade: string; }, any>; rules: { ...; }; render: ({ field: { value, onChange, onBlur } }: { ...; }) => Element; }' is not assignable to type 'IntrinsicAttributes & { render: ({ field, fieldState, formState, }: { field: ControllerRenderProps<{ cep: string; logradouro: string; numero: number; complemento: string; estado: string; bairro: string; cidade: string; }, "cep">; fieldState: ControllerFieldState; formState: UseFormStateReturn<...>; }) => ReactElemen...'.
Property 'as' does not exist on type 'IntrinsicAttributes & { render: ({ field, fieldState, formState, }: { field: ControllerRenderProps<{ cep: string; logradouro: string; numero: number; complemento: string; estado: string; bairro: string; cidade: string; }, "cep">; fieldState: ControllerFieldState; formState: UseFormStateReturn<...>; }) => ReactElemen...'.ts(2322)
Qual versão vc tá usando?
1 curtida
"@types/react-input-mask": "^3.0.5",
"react-input-mask": "^2.0.4",
"react": "18.2.0",
"react-redux": "8.0.5",
pode tirar o as={}
.
Ficando assim:
<Controller
name={'cep'}
control={control}
rules={{required: true}}
render={({field}) =>
<InputMask
{...field}
required
mask="99999-999"
placeholder={'CEP'}
defaultValue={user.cep}
/>
}
/>
1 curtida
<Controller
name='cpf'
control={control}
rules={{ required: true }}
render={({ field }) => <TextField {...field} required mask='999.999.999-99' placeholder={'CEP'} />}
/>
Tem que ser para o TextField. Como fazer ?
TextField é de alguma lib?
1 curtida
Qual versão?? Vi um InputBase aqui na v5, mas não sei se vc ta usando a mesma
1 curtida
Achei uma function que é melhor.
function FormattedInput(props: any) {
const {inputRef, ...other} = props;
return (
<InputMask
{...other}
ref={inputRef}
mask="999.999.999-99"
placeholder="CPF"
defaultValue={user.cep}
/>
);
}
return (
....
<Controller
name={'cpf'}
control={control}
rules={{required: true}}
render={({field}) =>
<TextField
required
{...field}
label={"CPF"}
InputProps={{
inputComponent: FormattedInput
}}
/>
}
/>
)
1 curtida
Acho que está parecido com o seu.
import InputMask from 'react-input-mask'
<FormControl fullWidth sx={{ mb: 4 }}>
<Controller
name='cpf'
control={control}
rules={{ required: true }}
render={({ field }) => (
<InputMask {...field} required mask='999.999.999-99' placeholder={'CPF'}>
<TextField label='CPF' />
</InputMask>
)}
/>
{errors.cpf && <FormHelperText sx={{ color: 'error.main' }}>{errors.cpf.message}</FormHelperText>}
</FormControl>
1 curtida